Integration Best Practices
EThe following is a detailed walk-through of the best practices and API methods to be used for an integration in which a Predictive Index (PI) assessment is initiated by an external system, usually an Applicant Tracking System (ATS), using an email message to invite candidates and an optional assessment status webhook to signal completion and then transfer results into the ATS. Along the way, various optional considerations are explained.
The following two sections and sequence diagrams represent the most common implementation of an API integration. Other implementations are of course possible, but it is the intent of this article to address the most commonly used strategies.
Part A - Triggered Email Invite, Assessment Completion Calls Webhook
Diagram A:
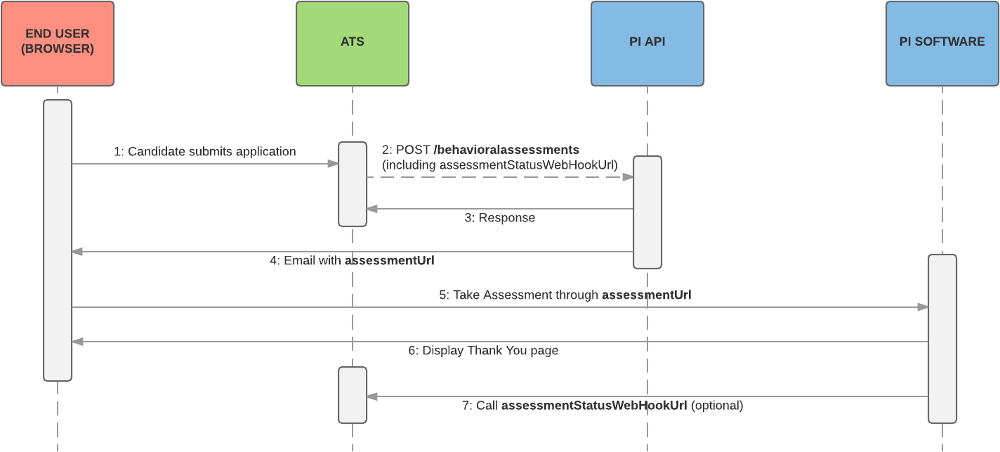
1. Candidate Selected for Assessment
A candidate typically is selected to take an assessment in an ATS when one of these occur:
- Candidate submits a completed online application for a job marked as requiring an assessment
- Recruiter manually performs an action within the ATS software to assign an assessment to a candidate
The PI platform does not automatically enforce upon an integration that each person is sent only one assessment if they apply for multiple jobs. It will allow your integration logic to create many duplicate persons with the same name and email address if this possibility is not considered. Avoiding duplication must be enforced by the integration program logic itself. When a candidate reaches a point where an assessment would be expected, the integration logic must first check for an existing assessment and decide whether to request a new assessment or use an existing one. Additional considerations may apply to your integration goals as outlined in the remainder of this section.
1a. Search for Existing Assessment Taker and Any Assessments
- Use the candidate's details to determine whether the candidate already has completed the required assessment(s)
-
- Existing ATS data referring to a previously completed assessment
- Existing assessment data in the PI system obtained through an API call using unique candidate data such as email address or candidate ID from the ATS system stored in the externalPersonId field. See GET peopleandjobs
GET /api/v2/peopleandjobs?$search=somename%40mymail.com
or
GET /api/v2/peopleandjobs?
$filter=peopleAndJobsSearchResult%2FassessmentUser%2FexternalPersonId%20eq%20'a75be8a5e5a5'
1b. Use Any Completed Assessment Results
If the candidate has already taken all required assessments, no new order should be required. Instead, the existing assessment data should be retrieved and stored according to the current integration design. The assessmentId values returned from the peopleandjobs call above can be used in any API methods relating to the assessment, and the assessmentUserId can be used in any API methods relating to the person.
If the candidate has an existing complete assessment but you will be using the Job Fit / Match Scoring features of PI, it may be necessary to update the existing person data with a current PI Job name so that the existing behavioral and cognitive assessment results will be compared with the correct PI Job rather than whatever PI Job may have been assigned previously. The API method to use for this PATCH v1\persons\{id}
Example using CURL (line breaks added):
curl -X PATCH --header 'Content-Type: application/json' --header 'Accept: application/json' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
-d '{ \ "associatedPositionTitle": "Programmer" \ }' 'https://pi.predictiveindex.com/api/v1/persons/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
1c. Resend Pending, Expired or Aborted Assessment Invitation
If the candidate has been previously assigned an assessment, there are various options for what to do next based on the assessmentState property of the existing assessment:
AssessmentState | Behavioral Assessment (BA) | Cognitive Assessment (CA) |
10 = Sent - an assessment was created. Leaving the assessment untouched will allow the person to complete it. | Resend = OK. If you wish to remind the person to take it, a PATCH behavioralassessments with parameter resendAssessment will send a new invitation without creating a new assessment. Software Note: If a user clicks "Send Again", another invitation for the same assessment will be emailed. |
Resend = BAD. If you wish to remind the person to take it, using either PATCH cognitiveassessments or POST cognitiveassessments will create a new assessment and disconnect the original assessment from the person. They can still follow an email link to the old assessment and complete it, but if a new assessment was sent, the old one will not be shown in the person results available via the API. Software Note: If a user clicks "Send New Assessment" or "Send Again", a new assessment will be sent and no optional properties such as externalId or assessmentStatusWebHook will be retained by the new assessment. Both old and new assessments will be available via the PI software, but not via the API. |
20 = Opened (CA only) - The invitation link was followed. | Not applicable | Resend = BAD. The assessment was not started, so it is still valid and can be started in the future, just like assessment state 10 = Sent. |
30 = Started (CA only) - The assessment taker began the timed assessment. | Not applicable | Resend = BAD. This status only exists while the assessment taker is actively working on an assessment. Any automated integration should leave such an assessment alone. |
40 = Complete | In general, the Self portion of a BA is accurate for years. If an existing completed assessment is available it can be used. If a some optional person properties such as associatedPositionTitle, folderPath, or personType have changed since it was completed, use PATCH persons to update the assessment taker data and then re-obtain results if they are affected by which PI Job is assigned, such as match scores or Interview Guides. If a BA is considered by the client to be too old, then use a DELETE behavioralassessments/{id} to archive it and order a new one using POST behavioralassessments for the same person using assessmentUserGuid parameter. Software Note: Multiple past BAs can be seen in the software by choosing a drop-down in the Behavioral block on the Person Overview page. |
In general, an automated integration should accept a completed CA. If a some optional person properties such as associatedPositionTitle, folderPath, or personType have changed since it was completed, use PATCH persons to update the assessment taker data and then re-obtain results if they are affected by which PI Job is assigned, such as match score. Only a human should determine whether a re-take is needed. If a re-take decided by a human is to be performed via the API, there is no way to use the API to obtain results of the original CA if a new CA is ordered for the same person. Only the latest CA is available via the API. So before ordering a new assessment using POST cognitiveassessments, be sure to store the result of the original assessment in an external system. Software Note: Multiple past CAs can be seen in the software by choosing a drop-down in the Cognitive block on the Person Overview page. |
50 = Expired - the assessment reached the expiration date without being completed. | Resend = GOOD. If you use PATCH behavioralassessments with resendAssessments and notifyAssessmentAvailableUsingEmail, the configured expiration date will be re-calculated and a new invitation sent. This preserves the other properties of the original assessment. Software Note: If a user clicks "Send Again", another invitation for the same assessment will be emailed after recalculating the expiration date. All optional properties are retained such as externalId and assessmentStatusWebHook. |
Resend = BAD; POST New = GOOD. Resending an existing CA does not work like the BA -- a new assessment is always created without transferring any of the optional properties from the original assessment and the result of a PATCH does not include the new assessmentId. Therefore, using a POST cognitiveassessments is best any time the CA is expired. Software Note: If a user clicks "Send New Assessment" or "Send Again", a new assessment will be sent and no optional properties such as externalId or assessmentStatusWebHook will be retained by the new assessment. |
60 = Failed 65 = Aborted (CA only) |
Not applicable | Resend = BAD; POST New = GOOD. These statuses are functionally equivalent to 50 = Expired and should be handled the same way. |
When using PATCH or POST methods detailed below to respond to expired or aborted assessments by sending new email invitations, it is often desirable to send an explanatory message to the email recipient explaining what is happening. To do this, define values for the following two properties in the JSON being passed:
- subject - The email subject line to be used in the new email invitation. If omitted, the original subject line will be re-used.
- message - The custom message to be included in the new email invitation. If omitted, the original message will be used.
PATCH behavioralassessments/{id} - To update an existing Behavioral assessment, the PATCH verb is used instead of the POST verb, the assessmentId must be passed as a part of the request URL and the body of the request must contain JSON with any properties to be updated. See PATCH behavioralassessment/{id}.
- resendAssessment - To resend the assessment invitation email, pass the parameter resendAssessment with a value of 1.
- expirationDateTime - Available only in the PATCH behavioralassessment method, use this to set a new expiration date (e.g. "2019-02-24"). If you want to specify a UTC time: "2019-02-24T04:00:00.000Z".
curl -X PATCH --header 'Content-Type: application/json' --header 'Accept: application/json' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
-d '{ "resendAssessment": true, "expirationDateTime":"2019-02-24", "subject":"The Predictive Index Behavioral Assessment - Extended", "message":"You have been given extra time to complete the Behavioral Assessment." }' 'https://pi.predictiveindex.com/api/v1/behavioralassessments/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
While it is possible to use PATCH cognitiveassessments with a resendAssessment parameter (as with the Behavioral assessment described above), this action will cause the existing Cognitive assessment to be archived and a new assessment created in its place, rendering the original Cognitive assessmentId invalid without transferring all of the optional fields from the original assessment to the new. In addition, PATCH does not return the new Cognitive assessmentId or any other response body. So using PATCH with resendAssessment may be functionally similar to POST, but the side effects are undesirable. Using POST is practically better because it returns the new assessmentId for subsequent use and it supports the passing of all optional parameters.
NOTE: When using POST cognitiveassessments to replace an existing one, be sure to pass optional parameters such as administeredBy*, externalId, notifyAssessmentAvailableUsingEmail, and assessmentStatusWebHook again because they are not copied over from the original assessment. You CAN use the same externalId value for the replacement assessment in this case without a uniqueness collision error because the original is archived before the new one is created.
curl -X POST --header 'Content-Type: application/json' --header 'Accept: application/json' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
-d '{ "assessmentUserGuid": "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx" "subject":"The Predictive Index Cognitive Assessment - Extended", "message":"You have been given extra time to complete the Cognitive Assessment." "assessmentStatusWebHook": "https://webhook.site/xxxx11114e46", \ "notifyAssessmentAvailableUsingEmail": 1, \ "externalId": "1234503002", \ }' 'https://pi.predictiveindex.com/api/v2/cognitiveassessments'
1d. Update Existing Person with New Details
Changing Job using Job Title (uses api/v1)
Maybe you have obtained a list of possible jobs from the /api/v3/jobs API method and stored them in a drop-down list in the UI and your UI doesn't support list values that are different from their display values, and now you wish to use the name property from that drop-down to assign the job to the current person.
curl -X PATCH --header 'Content-Type: application/json' --header 'Accept: application/json' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
-d '{ "associatedPositionTitle": "Quality Assurance Engineer" }' 'https://pi.predictiveindex.com/api/v1/persons/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
Changing Job using Job ID (uses api/v3)
Maybe you have obtained the list of possible jobs from the /api/v3/jobs API method and stored them in a drop-down list in the UI, and now you wish to use the jobId property from that drop-down to assign that jobId to the candidate in question.
curl -X PATCH --header 'Content-Type: application/json' --header 'Accept: application/json' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
-d '{ "associatedJobId": "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
}' 'https://pi.predictiveindex.com/api/v3/persons/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
Changing folderPath (uses api/v1)
Maybe the person is applying for a job in a different department than the previous application so you want to put their existing assessment information into a folder that is accessible by the hiring team for the latest application.
curl -X PATCH --header 'Content-Type: application/json' --header 'Accept: application/json' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx' -d '{ "folderPath": "\\Some Client Account\\Some Folder\\Another Folder" }' 'https://pi.predictiveindex.com/api/v1/persons/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
Changing externalPersonId (uses api/v3)
Maybe you need to update the external person ID because the existing data was created before you integrated with the current external system and need to assign an external person ID for the first time, or PI was integrated with a different external system previously so the new external person ID must be stored.
curl -X PATCH --header 'Content-Type: application/json' --header 'Accept: application/json' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
-d '{ \ "externalPersonId": "SOMEnewEXTID1234" \ }' 'https://pi.predictiveindex.com/api/v3/persons/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
1e. Example Logic Handling Existing Assessments along with Jobs and Folders
When dealing with a situation where a candidate applies for multiple jobs and the integration is designed to assign jobs and folders based on which job application is submitted, the integration logic must handle these changes. When the first assessment is ordered for the first job application, a folder will be chosen. When the second job application is completed and the integration logic decides what to do, it is an open question whether you want it to move the existing assessment/candidate to a new folder for the second job or to leave it in the original location. Either is a possibility, but each path requires different logic to be constructed and possibly different rules to govern the action.
For example, if the applicant applies for 4 jobs in one day, you might assume the first job they applied for is their top choice and the other three are "backup" jobs. This assumption would lead you to leave the assessment/person in the original (first) folder. On the other hand, if an applicant applies for one job and then a month later for a second job, you might assume the second job is now their top choice, leading you to move the assessment/person to the folder for the second job. To sort this out, your integration may need to include logic something like this:
if an assessment exists for this person (using email address to search) {
if assessmentDateTime (sent) is more than 30 days ago {
PATCH person: assign new job (associatedPositionTitle) assign new folder location (folderPath) corresponding to new job application
} else {
no change - leave assessment in original folder location with existing job
}
if the assessment is expired (assessmentState > 40) {
resend: BA = PATCH assessmentId + resendAssessment; CA = POST using assessmentUserId
}
} else {
POST a new assessment creating a new person with new job title and placed in new folder
}
2. ATS Integration Orders PI Assessment (POST)
When ordering an assessment via the PI API, you must first determine which API method to use based on which type of assessment you are ordering:
- Behavioral Assessment (aka BA or "the PI") = POST behavioralassessments
- Cognitive Assessment (aka CA or "the PLI") = POST cognitiveassessments
You must pass as the POST data a JSON object containing parameters that express several facts and decisions:
- Identify the Assessment Taker - there are two ways you can specify which person is supposed to take the assessment:
-
- Create a new PI person by providing new information about the person, causing a new PI Person object to be created along with the assessment. You cannot specify assessmentUserGuid if providing name and email data.
-
- firstName, middleName(optional), lastName
- externalPersonId(optional) - this is usually a unique identifier from the ATS system up to 71 characters in length. If you pass this value, an action that attempts to create a new PI Person, and that value already exists for another PI Person, you will receive an error and the POST will fail. This is why it is wise to check for an existing person in step 1 above before reaching this step.
- associatedPositionTitle(optional) - this field must match a Job Target name from the PI software. If this is defined as a parameter and the Job in PI software has a target defined for behavioral pattern and/or cognitive score, then the results of the assessment will include Job Match scores, a very powerful piece of data to use for identifying strong match candidates, even by people who do not yet fully understand the PI behavioral pattern graphs. To obtain a list of all current Job Target names, you can call the API method GET jobs and use from the results the selected name property to assign to associatedPositionTitle.
- gender(optional number or string) - Male (1) or Female (2) - controls the pronouns used in report language. If no gender is specified for a person, gender must be specified at the time Behavioral reports are generated (NOTE: The Behavioral Report is available in a gender-neutral form for en-US languageLocale only. See notes in Part B - Section 2 below.)
- personType (optional) - Candidate or Employee or Other. The PI software system includes an ability to manage persons and their assessment data according to the type of person they are to the organization. Setting the personType to Candidate for job applicants is wise so that all such people can be managed as a group. However, once a person has been hired, it is also wise to change their personType to Employee so they will no longer be managed like a Candidate.
- Refer to an existing PI person by providing the assessmentUserGuid of an existing PI Person to which the assessment should be linked. If you provide this parameter value, you cannot also provide values for the parameters used to define a new PI person as described above. Note: The value given to input parameter assessmentUserGuid should be the output property named assessmentUserId from the results of other API methods such as GET peopleandjobs or GET behavioralassessments, etc.
- Control whether and how the PI system notifies the Assessment Taker via email
-
- notifyAssessmentAvailableUsingEmail- 0 (No - default) or 1 (Yes) - If you wish the PI system to notify the user of the assessment, you may also wish to provide an email subject and introductory body content using the following two parameters:
- subject (optional) - email subject line to be used instead of the default value defined in the PI account settings.
- message (optional) - introduction shown at the very beginning of the email message body to be used instead of the default value defined in the PI account settings.
- Override the Assessment Owner and Contact Info - Assessments ordered via the API are automatically "owned" by the user account whose API Key is in use for the integration. See Generating an API Key for more details. The first and last name, email address, and phone of that user are the default person shown in the invitation email and assessment website as the contact point if assessment takers have any questions. That person is also shown as being responsible for the assessment when displayed in the PI software. If you wish to attribute the assessment to a different person -- a recruiter or hiring coordinator, for example -- you must specify any parameters you wish to be used in place of the default settings:
-
- administeredByFirstName, administeredByLastName
- administeredByEmail - must be used when name parameters are used
- administeredByPhone- optional
- administeredByCompanyName - required for Cognitive, not available for Behavioral
- Actions to Perform After Assessment
-
- assessmentStatusWebHook (optional) - define a listener URL on your integration system that will expect a POST of several attributes for each status change for a given assessment. Use this to signal your integration that the assessment is complete and ready for results to be read from the PI system. The attributes sent include assessmentId, externalId, assessmentState, and assessmentSource. See Assessment Status WebHooks for details
- notifyAssessmentAvailableUsingEmail (optional) - use this to cause the PI system to send an email invitation to the assessment taker containing a link to the assessment and instructions. This notification message is sent using default subject and introductory message text as defined in the client settings in the PI software unless you also specify the following override parameters:
-
- subject
- message
-
- autoSendPIReportToAssessmentTaker (optional, Behavioral only) - 0 (default) = No, 1 = Yes. Some clients wish to send a copy of the assessment results report directly to the assessment taker.
- sendCognitiveAssessmentOnCompletion (optional, Behavioral only) - 0 (default) = No, 1 = Yes. If you wish to have your assessment taker take both Behavioral and Cognitive assessments, pass this parameter in POST behavioralassessments to automatically trigger the sending of a Cognitive assessment email invitation after the assessment taker completes the Behavioral assessment. This parameter is only available in the POST behavioralassessment method.
WARNING: This feature does not cause a notification to be sent to the assessmentStatusWebHook upon completion of the Cognitive Assessment. If your integration requires a Cognitive webhook notification, do no tuse this parameter but instead make a separate call to POST cognitiveassessments to order it passing as a parameter the assessmentUserId obtained from the response of the POST behavioralassessments call. - redirectURL (optional, Behavioral only) - a web address to which the assessment taker's browser should be redirected after completing the assessment. This can be used, for example, to show the user a web page explaining next steps. Or if the assessment is being presented to the assessment taker as part of the application process, a redirect might be used to take the user back to the next stage of the online application.
- Other Properties
-
- externalId (optional) - This field provides an opportunity to store a unique identifier with the assessment being ordered up to 71 characters in length. This is different from externalPersonId mentioned above which is associated with the person. If an externalId is defined for an assessment and an assessmentStatusWebHook URL has been defined, this value is included in the POST variables sent from the PI system to the listener at that URL.
- folderPath or folderGuid (optional) - The PI system always stores assessment takers into a folder within a structure controlled by the client. The default folder is determined on a client account level and can be overridden by a setting in the user account who owns the API Key. Those folder settings are ignored if a different folder is specified using folder-related parameters. Since permissions can be attached to folders by client admins, the owner of the API Key used by the integration must have sufficient permissions to access the specified folder. There are two ways to specify a folder into which an assessment taker should be placed, but you can only specify one or the other of these two:
-
- folderPath - a full path to the folder as displayed in the details area of the chosen folder within the PI system settings. Backslashes in the path must be doubled to escape them for use in JSON: "\\Example Company\\Some Folder"
- folderGuid - the PI system GUID for the given folder. This parameter may be used to avoid errors caused by spelling changes in the folder names.
- languageLocale (optional) - PI system will assume that the language for assessments is the same as the default language for the client account. Possible language codes are found here: API Guide - Common
- customInfo (optional) - an array of fieldName and fieldValue pairs containing any values you choose. WARNING: These fields are deprecated and may not be supported in future versions of the PI software and API.
2a. PI Sending Email
2b. ATS Sending Email
2c. Example POST behavioralassessments
curl -X POST --header 'Content-Type: application/json' --header 'Accept: application/json' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx' -d '{ \ "firstName": "Kim", \ "lastName": "Prospect", \ "email": "kim.prospect%40gmail.com", \ "subject": "ACME Corp - Please take Behavioral Assessment", \ "message": "As a part of your job application process, ACME Corp would like you to take this brief assessment to help complete our understanding of you! It only takes about 8 minutes.", \ "administeredByEmail": "trecruiter%40acme.com", \ "administeredByPhone": "800-555-1212", \ "administeredByFirstName": "Terry", \ "administeredByLastName": "Recruiter", \ "associatedPositionTitle": "Sales Manager", \ "gender": "Female", \ "personType": "Candidate", \ "notifyAssessmentAvailableUsingEmail": 1, \ "externalId": "A123456", \ "externalPersonId": "P345678", \ "folderPath": "\\ACME Corp\\Candidates", \ "languageLocale": "en-US", \ "assessmentStatusWebHook": "https://someintegrationserver.com/listener/pi-assessment", \ "redirectURL": "http://acme.com/careers/next-steps", \ "autoSendPIReportToAssessmentTaker": 1 \ }' 'https://pi.predictiveindex.com/api/v1/behavioralassessments'
Example of Response (HTTP response code 201):
{ "errorResponse": null, "assessmentId": "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx", "firstName": "Kim", "middleName": null, "lastName": "Prospect", "email": "kim.prospect@gmail.com", "impression": 0, "subject": "ACME Corp - Please take Behavioral Assessment", "message": "As a part of your job application process, ACME Corp would like you to take this brief assessment to help complete our understanding of you! It only takes about 8 minutes.", "assessmentDateTime": "2018-08-15T19:49:27.6886775Z", "expirationDateTime": "2018-08-23T12:00:00.0000000Z", "assessmentCompletedDateTime": null, "administeredByEmail": "trecruiter@acme.com", "administeredByPhone": "800-555-1212", "administeredByFirstName": "Terry", "administeredByLastName": "Recruiter", "scoringMethod": 1, "patternNumber": null, "descriptiveReportUrl": "", "associatedPositionTitle": "Marketing Director", "associatedPositionImageUrl": "/0?format=PNG", "folderId": null, "folderPath": "\\ACME Corp\\Candidates", "patternFullUrl": "", "patternSelfUrl": "", "gender": 2, "personType": "Candidate", "isDeleted": 0, "assessmentState": 10, "assessmentStatusWebHook": "https://someintegrationserver.com/listener/pi-assessment", "notifyAssessmentAvailableUsingEmail": 1, "externalId": "A123456", "externalPersonId": "P345678", "assessmentUserId": "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx", "assessmentUrl": "https://assess.predictiveindex.com/Account/LoginPi?a=XXXXXXXX8igigYxTzbfZQzR3Eeq2vrjn3xg2EesVjZuRWLScteGbnQQNVyKa20Kn&lang=en-US", "assessmentPassword": "XXXXXXXX", "descriptiveReportPageUrl": "", "personPageUrl": "https://pi.predictiveindex.com/Browse/PersonDetails?assessmentUserId=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx", "redirectURL": "http://acme.com/careers/next-steps", "selfSigmaScore": null, "selfConceptSigmaScore": null, "synthesisSigmaScore": null, "positionSigmaScore": null, "customFields": null, "customReports": null, "latestWebhookStatus": null, "autoSendPIReportToAssessmentTaker": 1, "sendCognitiveAssessmentOnCompletion": 0 }
3. ATS Integration Receives Response from POST
- assessmentUrl - the link URL for the assessment to be taken. This is included in the email sent from PI if that option is chosen. It can be used to display to the assessment taker or included in an email sent from the ATS software. It may also be stored in the ATS for use in recovering from a lost invitation email, though the link will not function after the assessment is completed.
- assessmentId - the internal PI reference to the newly created assessment. The integration logic may store this value for troubleshooting or future API calls.
- personPageUrl - the web address of the Person Detail page for the newly created or referenced PI person. Before the assessment is complete, this page will show the current assessment status. After the assessment is complete, results can be found here. This is the most commonly stored URL for candidates in ATS data.
- descriptiveReportPageUrl - the web address of the Report for this assessment. It is useful only after the assessment is complete and can also be reached from the personPageUrl location.
- See the POST behavioralassessments API Referencefor additional response properties.
4. Candidate Receives Assessment Email
- PI System Sends Email - if the parameter notifyAssessmentAvailableUsingEmail is set to 1, then the PI system will send an email message to the assessment taker containing instructions and a link. The following parameters, explained in section 2 above, control several aspects of this message:
- Subject - The email subject line will use the value of the subject parameter, if included, otherwise it will use the default in PI Software > General Settings
- Message - The introductory paragraph of the message body will use the value of the message parameter, if included, otherwise to be used in place of the default in PI Software > General Settings
- Sender - the First and Last name specified in the administeredBy parameters will show as the sender, if included, otherwise the contact details of the user who owns the API Key will show as sender
- Contact info - in the body of the message, contact info is provided to the assessment taker using administeredBy info, otherwise the contact details of the API Key owner will be used
- Language - the language used for the invitation will be determined by the languageLocale parameter passed in the assessment order, otherwise the default language locale as defined in PI Software > Administration > User Management > the Default Language preference of the user who is the owner of the API Key will be used.
- Integration System or Software Sends Email - if the parameter notifyAssessmentAvailableUsingEmail is set to 0, then the PI system will NOT send an email message to the assessment taker. The integration system or the non-PI software must display or send the assessmentUrl to the assessment taker.
5. Candidate Follows Link and Takes PI Assessment
The link whose value is defined in response property assessmentUrl is encoded to include a unique assessment identifier, a username, and a password that will allow immediate access to the assessment. Once the assessment is complete, the link cannot be used again.
6. Assessment End
7. PI Optionally Calls ATS Integration Assessment Status Webhook Listener
See Webhooks for more detail on how they work.
If the assessmentState is 40 (Complete), the next step would be to use the assessmentId in a call to GET behavioralassessments/{id} and/or possibly other methods to obtain all result details, as described in Part B:
Part B - Obtain Assessment Results Link to Additional Results
Diagram B:
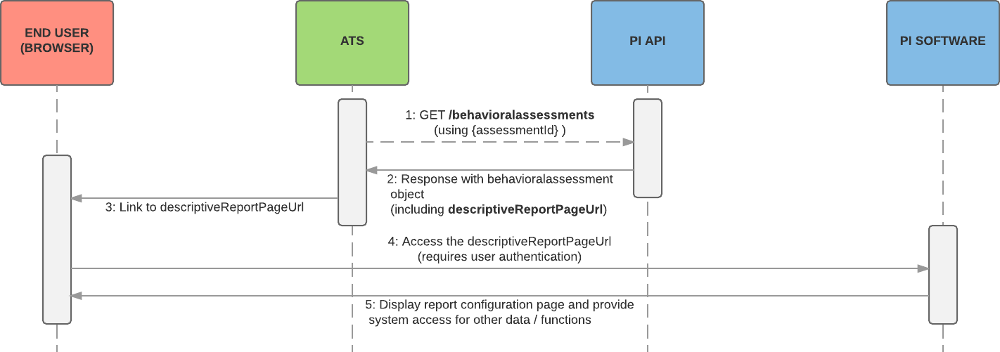
1. Obtain Assessment Results (GET)
- Behavioral Assessment: GET behavioralassessments/{assessmentId}
- Cognitive Assessment: GET cognitiveassessments/{assessmentId}
curl -X GET --header 'Accept: application/json' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
'https://pi.predictiveindex.com/api/v1/behavioralassessments/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
2. Receive and Store Assessment Details
Behavioral Assessment Results - GET behavioralassessments/{assessmentId}
- assessmentStatus - this numerical value is usually converted into some text string that makes sense to end users, such as "Sent" or "Complete". The meanings of these numbers is defined here: API Guide - Common
- personPageUrl - this is a web browser-friendly URL pointing to the PI software page which contains everything known about the assessment taker including results and reports. If nothing else is stored in the ATS as an assessment result, this link is probably the most useful item. Keep in mind that any link pointing to the PI software will require the user to log into the PI system. If your ATS users are not in possession of PI software user credentials, this will not work for those users. So you must either grant them PI software access or use another method of making PI results available, described below.
- descriptiveReportPageUrl - this is a web browser-friendly URL pointing to the PI software page containing the Behavioral Report for the assessment taker. This is probably the second most valuable link (after personPageUrl) to display for users. Clicking this link does not immediately show a PDF Report, however.
- descriptiveReportUrl - this is an API method call, NOT a web browser-friendly link. You cannot simply display this link and expect users to click on it. Instead, you can use this link to make a secondary API method call which will return as a result the actual Behavioral Report PDF file. If you call this URL as it is delivered in the response property, you will get a response of a ZIP file containing two reports using Male and Female pronouns. In most cases, it is more convenient to your end users if you obtain a single PDF file, even if you have to use a default gender pronoun selection. (NOTE: Gender-neutral reports are currently available only for en-US languageLocale. To specify gender-neutral, add the gender parameter with no value "gender=") To get a single PDF file as a response, you must add a gender parameter to specify the pronouns to use, using either the number or string representation (Male=1, Female=2).
https://pi.predictiveindex.com/api/v1/behavioralassessments/{id}/1?format=PDF&gender=Female
You must also choose how to deliver this PDF file. There are two general strategies used: -
- Store PDF in ATS - some ATS systems support attaching files to the candidate profile or the assessment results data structures. This is probably the most desirable because it avoids reliance on the integration and creates a more instantaneous and permanent access opportunity.
- Stream PDF from PI to Browser - if the ATS system cannot allow PDF file upload, you can construct a web request handler in your integration solution that will take GET requests coming from a link you build and insert into the ATS data/UI which convert the request into an API method call that gets the PDF and streams it back to the browser. In this way, the end-user clicks a link and a PDF file pops up. This solution may be slower than storing the PDF file in the ATS system, but it makes report results available without the user having to log into PI and requires zero file storage in the ATS.
- Also see the notes below in Downloading Reports regarding multiple methods available to download reports in PDF format.
curl -X GET --header 'Accept: application/json' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx' 'https://pi.predictiveindex.com/api/v1/behavioralassessments/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
Reference Pattern Details - GET behavioralassessments/{assessmentId}/guidebook
- referencePattern/name, /description, /traits - these are good summary facts to display for an assessment taker
curl -X GET --header 'Accept: application/json' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx' 'https://pi.predictiveindex.com/api/v2/behavioralassessments/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx/guidebook?languageLocale=en-US'
Note: Reference Pattern name, description, and icon URL (but not traits) are also available from the GET peopleandjobs/{assessmentUserId} method.
Cognitive Assessment Results - GET cognitiveassessments/{assessmentId}
- assessmentState - like the Behavioral assessment, displaying the current status of the assessment is often desirable. Be sure to convert numeric values into something human-readable. The meanings of these numbers is defined here:API Guide - Common
- score - the value from 100 to 450 representing the assessment score - this is the score displayed within the PI software as the Cognitive score and in Cognitive reports
- correct - the value from 0 to 50 representing the number of questions answered correctly out of 50
- percentileRank - a value from 1 to 100 representing the estimated position in the population of assessment takers with 1 being the lowest score and 100 being the highest score. A score of 92 indicates that 92% of assessment takers score the same as or lower than this result.
- descriptiveReportUrl - an API method call URL that can be used to retrieve the PDF report file for this cognitive result. See the comments regarding options for handling the Behavioral assessment result property of the same name, above.
curl -X GET --header 'Accept: application/json' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx' 'https://pi.predictiveindex.com/api/v2/cognitiveassessments/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
Job Match Score - GET persons/{assessmentUserId}
- behavioralJobFitScore - a whole number 1-10 representing the strength of the match to the target pattern
- cognitiveJobFitScore - a whole number 1-10 representing the strength of the match to the target score
- combinedJobFitScore - a floating point value from 1-10 representing the combination of the other two scores using the weighting factor defined in the PI software. Example: 5, 7.5, 8.25, or 9
curl -X GET --header 'Accept: application/json' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx' 'https://pi.predictiveindex.com/api/v1/persons/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
Note: Job Match Scores are also available from the GET peopleandjobs/{assessmentUserId} method.
Downloading Reports
- *assessments method reports (using GET)
- persons method reports (using POST)
Desired Report | Persons API (POST) | *assessments APIs (GET) |
Behavioral Report | /v1/persons/PrintBehavioralReports | /v1/behavioralassessments/{id}/reports/1 |
Cognitive Report | /v3/persons/PrintCognitiveReports | /v2/cognitiveassessments/{id}/reports/0 |
PrintBehavioralReports
Person ID - the personId parameter is a PI assessmentUserId value explained earlier in this article.
Gender Note: When reportLanguage = "en-US", you may define the gender = Unknown in order to receive a gender-neutral report. All other reportLanguage values must specify a gender (Male, Female).
Selling/Influencing Note: The two flags "isSellingStyle" and "isInfluencingStyle" are mutually exclusive which means only one will be included in the report even if you specify "true" for both. The difference is that the "Selling" content uses terminology specific to sales processes and "Influencing" content uses more general language.
curl -X POST --header 'Content-Type: application/json' --header 'Accept: application/pdf' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx' -d '{
"reportLanguage": "en-US", "personsToReportOn": [ { "personId": "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
"gender": "Unknown" } ], "isManagementStyle": true, "isSellingStyle": true, "isInfluencingStyle": false }' 'https://pi.predictiveindex.com/api/v1/persons/PrintBehavioralReports'
PrintCognitiveReports
curl -X POST --header 'Content-Type: application/json' --header 'Accept: application/pdf' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx' -d '{ "reportLanguage": "en-US", "personsToReportOn": [ "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx" ], "showSensitiveInformation": false }' 'https://pi.predictiveindex.com/api/v3/persons/PrintCognitiveReports'
Job Interview Guide - POST persons/DownloadInterviewGuide
curl -X GET --header 'Accept: application/json' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx' 'https://pi.predictiveindex.com/api/v2/peopleandjobs/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
curl -X POST --header 'Content-Type: application/json' --header 'Accept: application/pdf' --header 'api-key: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx' -d '{ "personId": "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx", "jobId": "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx", "guideLanguage": "en-US" }' 'https://pi.predictiveindex.com/api/v3/persons/DownloadInterviewGuide'
Person Snapshot Report - GET persons/downloadPersonSnapshotReport
The Person Snapshot report is a useful summary of a person's behavioral pattern intended for sharing with others who may not have any PI training or understanding. To obtain this report, pass an assessmentUserId in the parameter personId, a filename (without the file extension), and languageLocale (optional) in an API call like this (line breaks added to what should be one single URL):
curl -X GET --header 'Accept: application/pdf' --header 'api-key: e8c11cd0-4be7-45ce-b1fe-2a051373de75' 'https://pi.predictiveindex.com/api/v3/persons/downloadPersonSnapshotReport?personId=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx&fileName=TestPersonPIBehavioralSnapshot&languageLocale=en-US'
The filename passed as a parameter will have a ".pdf" extension added to the response file stream.